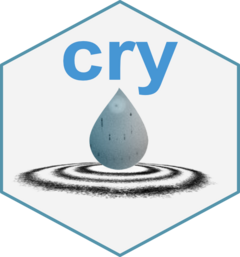
Calculation of structure factors
strufac.Rd
Calculation of structure factors for any input reflection
Arguments
- hkl
An m X 3 array or data frame of integers. Each row is a set of Miller indices, \((h,k,l)\).
- xyzf
An n X 3 array or data frame whose rows are the fractional coordinates of the n atoms in the asymmetric unit.
- aname
A character string. The name of the atomic species.
- B
A vector of length n providing the thermal factor for the n atoms in the asymmetric unit.
- Occ
A vector of length n providing the occupancy numbers for the n atoms in the asymmetric unit.
- SG
A character string indicating the extended Hermann-Mauguin symbol for the space group.
- cpars
A length-6 vector containing the unit cell parameters. The first three numbers are a, b, c, the last three are the angles \(\alpha, \beta, \gamma\) in degrees.
Value
A named list with amplitudes (name "F") and phases in degrees (name "phi") of the structure factors calculated. Each component of the list is a vector of length equal to the number of input Miller indices.
Details
The theoretical formula in P 1 for the calculation of a structure factor with Miller indices \((h,k,l)\) is, $$ F(h,k,l) = \sum_{j=1}^N Occ_j f_j(s)\exp(-B_j s^2/4) \exp(2\pi\mathrm{i}(hx_j+ky_j+lz_j)) $$ where \(B_j\) is the B factor for atom j, \(s=|\mathbf{h}|\), \(f_j\) is the scattering factor for atom j and \(Occ_j\) is the occupancy for atom j. When a symmetry is present, only the atoms in the asymmetric unit are necessary as they are expanded into a P 1 structure using symmetry operators. The specific symmetry will be reflected in amplitude and phase of specific structure factors having precise values (e.g. 0, 90, 180, etc) or being zero (systematic absences).
Examples
# Random 5-atom structure in P 21 21 21
# Asymmetric unit is 0 < x < 1/4, 0 < y < 1, 0 < z < 1
xyzf <- matrix(c(runif(5,min=0,max=0.25),
runif(5,min=0,max=1),
runif(5,min=0,max=1)),ncol=3)
# Atoms are C, N, O, F, Fe
aname <- c("C","N","O","F","Fe")
# Random B factors around 1
B <- rnorm(5,mean=1,sd=0.5)
# No atoms in special position
Occ <- rep(1,times=5)
# Space group xHM symbol
SG <- "P 21 21 21"
# Cell parameters
cpars <- c(20,30,40,90,90,90)
# Create a few Miller indices
hkl <- expand.grid(h=-2:2,k=-2:2,l=-2:2)
# Creation of structure factors
lSF <- strufac(hkl,xyzf,aname,B,Occ,SG,cpars)
# Check systematic absences (give lSF$F = 0)
# For P 21 21 21 they are:
# (h,0,0),h=2n
# (0,k,0),k=2n
# (0,0,l),l=2n
fullidx <- 1:125
jdx <- sysabs(hkl,SG)
idx <- fullidx[-jdx] # These are the systematic absences
print(hkl[idx,])
#> h k l
#> 38 0 0 -1
#> 58 0 -1 0
#> 62 -1 0 0
#> 64 1 0 0
#> 68 0 1 0
#> 88 0 0 1
print(lSF$F[idx])
#> [1] 0 0 0 0 0 0
# Now check reflections with (h,k,0). h even has phase 0,180
# and h odd has phase 90,270
idx <- which(hkl[,3] == 0)
print(lSF$phi[idx])
#> [1] 1.242901e-14 9.000000e+01 1.800000e+02 9.000000e+01 8.078859e-14
#> [6] 1.800000e+02 9.000000e+01 0.000000e+00 2.700000e+02 1.361093e-13
#> [11] 1.800000e+02 0.000000e+00 0.000000e+00 0.000000e+00 1.800000e+02
#> [16] 3.600000e+02 9.000000e+01 0.000000e+00 2.700000e+02 1.800000e+02
#> [21] 3.600000e+02 2.700000e+02 1.800000e+02 2.700000e+02 3.600000e+02